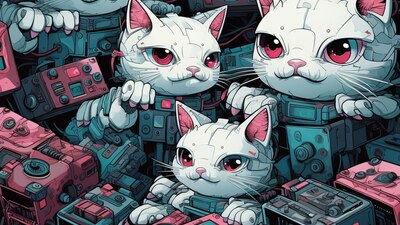
Models | Ruby on Rails
Basics of models. Communication between models. Validation and callbacks
Views are the part of a web application that is responsible for how information is displayed to users on a page. It defines how the data will look and how it will be structured. In Ruby on Rails, views are created using templates that help design the page.
The purpose of this article is to explain and demonstrate key concepts related to views in Ruby on Rails . We'll look at how to create views, how they interact with other components of a web application, and how they help developers create stylish and intuitive web pages. For better understanding, we'll also provide code examples and illustrations to show how it all works in practice. So, let's move on and learn more about how Ruby on Rails helps us manage views, create structured pages, and give them a user-friendly look.
The main purpose of views in Ruby on Rails is to separate the logic of the application and the appearance of the pages. Views are responsible for how data is interpreted and displayed on a web page. They take the form of HTML templates in which you can embed dynamic data. It helps to create very dynamic and interactive pages.
Ruby on Rails supports several different templating languages, and one popular one is Slim . Slim is a minimalist template language that allows you to write HTML code more compactly and clearly. It is ideal for working with views in Rails , as it allows you to quickly create structured and understandable templates.
In addition to Slim , Ruby on Rails supports other patterns such as ERB (Embedded Ruby), Haml , and many others. Choosing a template is a matter of the developer's taste, and everyone can choose the one they like best and suit their needs. I personally use Slim on my projects.
In Rails , the view directory structure is pretty standard. It is usually located in the folder. Each controller has its own folder with a name that corresponds to the name of the controller. For example, if you have a controller named ArticlesController , then the corresponding views are stored in the folder app/views/articles
.
Each view folder can have different templates for different controller actions. For example, for the show action , the template will have the name show.html.slim . For index action - index.html.slim and so on. This framework helps Rails automatically determine which view to use for each controller action.
This way, you can create structured and organized views for your Ruby on Rails application . In the following parts of the article, we will take a closer look at how to create these views and how they interact with other components of the application.
In the context of Ruby on Rails , layouts are special templates that allow you to define the general structure and appearance of pages for the entire application or for a specific controller. Layouts define the header, navigation, styles, JavaScript , and other elements that are repeated across all pages.
Layouts help avoid code duplication and make your app more organized and easy to change. They allow developers to set common design elements, such as a navigation menu or a footer, in one place and use them on all pages.
Creating a layout in Ruby on Rails is a simple task. You can create a new layout in the folder . For example, let's create a layout with the name app/views/layouts
.
Here is an example of a simple layout in a file: application.html.slim
html
head
title My Awesome Rails App
= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track' => true
= javascript_include_tag 'application', 'data-turbolinks-track' => true
body
= yield
A Layout has a page header, style bindings and JavaScript , and main content defined by the yield keyword . All content from controller actions will be inserted into this location.
To use this layout for a specific controller, you need to specify it in the controller. Example:
class ArticlesController < ApplicationController
layout "application" # Specify to use layout "application" for this controller
# ...
end
How layouts make a developer's life easier
Layouts greatly simplify the development and maintenance of web applications. Here are a few ways to explain why they are so important:
Ensure unity of appearance : You can ensure unity of appearance for the entire application or for a specific controller, making it more professional and easily recognizable to users.
Avoid code duplication : You can put common code, such as a navigation menu, in just one place to avoid duplication and make changes quick and easy.
Ease of making changes : If you want to change the look of the entire application, you only have to make changes in one place - the layout . This makes the redesign process faster and less error-prone.
As such, layouts are a powerful tool in Ruby on Rails that help developers manage the appearance of pages and maintain clean and organized web application code. In the following parts of the article, we will consider another important aspect of displays - rendering , which allows you to insert data into the page.
Ruby on Rails uses the render and render partial methods to display information on a page .
The render method is used to display the entire view . It tells Rails which view to use for a particular controller action. For example, you have a show action in the Articles controller , and you want to display information about a specific article. For this you use:
def show
@article = Article.find(params[:id])
render :show
end
The render method tells Rails to use a view show.html.slim
to render the page.
render partial , on the other hand, is used to display a portion of a view that can be embedded in other pages. For example, if you have a block with a list of articles and you want to display it on several different pages, then you use render partial :
# Displays the list of articles on the 'index' page
def index
@articles = Article . all
end
# app/views/articles/index.html.slim
h1 Articles
= render partial: 'article', collection: @articles
# app/views/articles/_article.html.slim
.article
h2 = article.title
p = article.content
In this example, the render partial uses a template for each article in the @articles collection, inserting them into the page _article.html.slim
.
To pass variables and data to a view in Ruby on Rails , you can use controller instance variables. For example, in the show controller action we discussed earlier, we pass the @article variable to the view:
def show
@article = Article.find(params[:id])
render :show
end
In the view , you can access this variable: show.html.slim
h1 = @article . title
p = @article . content
This allows you to display information from the database on the page.
Here are some examples of using render and render partial :
Displaying a list of articles on the index page :
def index
@articles = Article.all
end
# app/views/articles/index.html.slim
h1 Articles= render partial:'article',collection:@articles
Displaying the form for creating a new article:
def new
@article = Article . new
end
# app/views/articles/new.html.slim
h1 New Article
= render 'form'
In this case, will display the form, which is in a separate template render 'form'
These examples illustrate how Ruby on Rails implements rendering and passing data to views, which helps create dynamic and interactive pages for web applications.
The correct design and appearance of pages is an important aspect of web application development. Users' first impression is how your app looks and how easily they can interact with it. Using views, layouts , and rendering in Ruby on Rails helps make your application more attractive and functional. By establishing the right structure and using templates, you can make changes and add new functionality more easily and quickly.
If you want to learn more about views, layouts , and rendering in Ruby on Rails , here are some useful resources for further study:
Book " Agile Web Development with Rails " - this book contains detailed information about working with mappings and templates in Ruby on Rails .
Ruby on Rails courses on learning platforms such as Coursera , Udemy , and Codecademy , which offer interactive lessons and assignments.
Knowledge of views , layouts , and rendering is an important part of learning Ruby on Rails and developing web applications. They help you create stylish and functional pages that will satisfy the needs of users and make your app more attractive. I wish you success in further study and development!