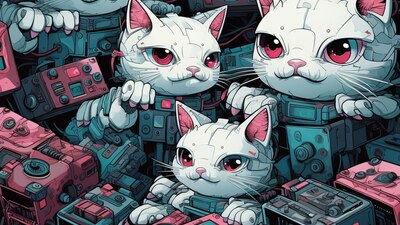
Models | Ruby on Rails
Basics of models. Communication between models. Validation and callbacks
Controllers in Ruby on Rails are a component of the MVC (Model-View-Controller) architectural pattern, used for building web applications. They are responsible for handling user input requests and interacting with models and views. Controllers contain methods called "actions," and each action handles a specific type of request.
To create controllers in Rails, you use a generator. It will automatically create the necessary files and structure for your controller. The command to create a controller looks like this:
rails generate controller ControllerName
Replace "ControllerName" with the desired name of your controller.
Controllers are typically located in the "app/controllers" directory in your Rails project. Each controller is a class that inherits from the ApplicationController class. For example:
class UsersController < ApplicationController
# controller code goes here
end
Controllers can have different actions that respond to different requests from users. Common actions include index, show, new, edit, create, update, destroy, and others. Action names are often used in routes to determine which action should be performed for a specific URL.
Controller actions can receive parameters from the URL. For example, if you have an action that takes a user's id from the URL, you can access this parameter as follows:
class UsersController < ApplicationController
def show
user_id = params[:id]
# do something with user_id
end
end
Every controller action should return a response to the request. Typically, this involves rendering a view that displays a page for the user. For example:
class UsersController < ApplicationController
def index
@users = User.all
# render the index view
end
end
Variables defined in a controller action can be used in the corresponding view. For example, if we define a @user variable in the index action, we can display it in the index view as follows:
#/ app/views/users/index.slim
h1 Users
ul
- @users.each do |user|
li = user.name
Filters allow you to execute code before or after executing controller actions. For example, you may need to check if a user has access rights to the controller actions before executing them. Filters help keep your code clean and organized.
Let's say you need to check if the user has permission to edit a certain record before performing the "edit" action:
# app/controllers/posts_controller.rb
class PostsController < ApplicationController
before_action :authorize_user, only: [:edit]
def edit
@post = Post.find(params[:id])
end
private
def authorize_user
# access rights checking code here
# for example, check if the user is the owner of the post
unless current_user && current_user == Post.find(params[:id]).user
redirect_to root_path, alert: "You do not have the permission to edit this post"
end
end
end
In Ruby on Rails, there is an approach called "resourceful routes" that allows you to create standard routes for controllers that correspond to CRUD operations (create, read, update, delete) on resources in your application.
To use resourceful routes, define the route in the "config/routes.rb" file as follows:
resources :controller_name
Replace "controller_name" with the name of your controller. For example, if you have a controller for users, you can use the following code:
resources :users
Rails will automatically generate standard routes for this controller, including URLs for the list of users, viewing a single user, creating a user, updating, and deleting.
Controllers in Ruby on Rails play a key role in interacting with users and handling incoming requests. They allow you to keep the logic of interacting with data in separate components, making your code more organized and easier to understand.
In the section about controllers, we discussed creating controllers, actions, parameters, using variables in views, filters, and resource routes. Hopefully, this article will help you better understand and feel more confident in developing with Ruby on Rails. Keep learning and good luck on your programming journey! 😊