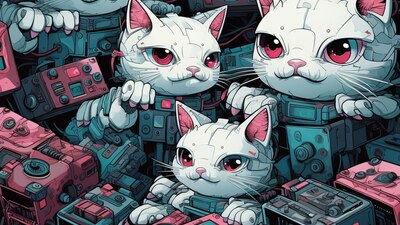
Models | Ruby on Rails
Basics of models. Communication between models. Validation and callbacks
In Ruby on Rails, routes play a crucial role in how your application handles HTTP requests from users. They help direct requests to the appropriate controllers and actions, establishing the connection between URLs and the functionality of the application.
In Ruby on Rails, routes are defined in the file config/routes.rb. They not only specify which controller actions correspond to different URL paths, but also allow the use of parameters, resource creation, and redirection.
In Rails, routes are defined in the config/routes.rb file. They include the URL path, HTTP methods, and references to controllers and actions.
Each route can be associated with HTTP methods such as GET, POST, PUT, DELETE. These methods lead to the execution of specific actions in the controller. For example:
# config/routes.rb
resources :articles
get '/about', to: 'static#about'
Parameters are defined as parts of the URL path that start with a colon. They are used to pass additional data to controller actions. For example:
get '/articles/:id', to: 'articles#show'
Simple path is a fixed URL without parameters. It is used to define static pages and controller actions that do not require parameter passing.
get '/about', to: 'static#about'
Simple paths are convenient to use for pages like "About Us", "Contact", "Terms of Use", and other static pages of your website.
Dynamic paths contain parameters that can vary for each request. They are used to identify resources or transmit additional information.
get '/articles/:id', to: 'articles#show', as: :article
Dynamic parameters allow defining URLs like /articles/1, /articles/2, and accessing the respective resources by their identifier.
Resource routes are a way to automate the creation of standard routes for CRUD operations (create, read, update, delete) over resources.
resources :articles
Resource routes help reduce repetitive code and create a convenient and standard way of working with resources.
Redirects are defined to redirect users to another URL after performing a certain action.
get '/old-url', to: redirect('/new-url')
Redirects can be temporary (HTTP 302) or permanent (HTTP 301), depending on the application's needs.
# config/routes.rb
get '/about', to: 'static#about'
In this example, we defined a simple route /about that leads to the about action of the Static controller.
# config/routes.rb
get '/articles/:id', to: 'articles#show'
This route uses the :id parameter, which will be used to determine the article to display.
# config/routes.rb
resources :articles
This single line will create all the standard routes for the articles resource, including index, show, new, create, edit, update, and destroy.
Routes in Ruby on Rails are a powerful tool for defining and organizing routes in your application. They help facilitate structured interaction between users and your application. Understanding how routes work is important for any Ruby on Rails developer, and they open up endless possibilities for creating dynamic and powerful web applications.