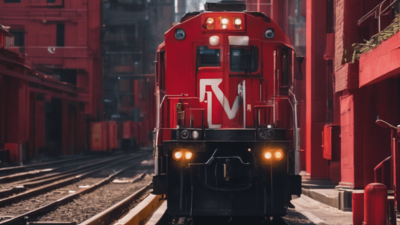
Моделі (Практика) | Ruby on Rails
Практичне завдання до теми в Моделі
Створити моделі Author(name) та Post(title, description, author_id). Створити PostsController з двома діями (actions): index та show. Для цих дій також створити шляхи та відповідні представлення. На сторінці index відображати всі пости, а на сторінці show - окремий обраний пост. Створити AuthorsController з діями index та show. На сторінці index цього контролера виводьте усіх авторів, а на сторінці show - інформацію про конкретного автора та його пости. Забезпечте можливість переходу на сторінку автора зі сторінки поста.
Створимо таблицю authors в БД.
rails generate model Author name:string
class CreateAuthors < ActiveRecord::Migration[7.1] def change create_table :authors do |t| t.string :name t.timestamps end endend
Створимо таблицю posts в БД.
rails generate model Post title:string description:text author:references{foreign_key:true}
class CreatePosts < ActiveRecord::Migration[7.1] def change create_table :posts do |t| t.string :title t.text :description t.references :author, foreign_key: true t.timestamps end endend
Давайте тепер встановимо наш зв'язок "один до багатьох" для наших моделей.
class Post < ApplicationRecord belongs_to :authorend
class Author < ApplicationRecord has_many :postsend
Створимо PostsController з діями index та show.
class PostsController < ApplicationController def index @posts = Post.all end def show @post = Post.find(params[:id]) endend
Виводимо в index усі пости з БД, в show окремий post за id.
Для цих дій створим шляхи.
Rails.application.routes.draw do root "posts#index" resources :posts, only: %i[index show]end
Після того нам потрібні відповідні представлення для дій index та show. Виводим список постів на сторінці index.
# app/views/posts/index.html.slimh1 Posts= render partial: 'post', collection: @posts
# app/views/posts/_post.html.slim.post h1 = post.title p = post.description h5 = post.author.name = link_to 'Переглянути', post_path(post.id)
У представленні show.html.slim, ми можем отримати доступ до окремого поста.
# app/views/posts/show.html.slimh1 = @post.titlep = @post.description= link_to 'Усі публікації', posts_path
Створимо AuthorsController з діями index та show.
class AuthorsController < ApplicationController def index @authors = Author.all end def show @author = Author.find(params[:id]) @posts = @author.posts endend
Виводимо в index усіх авторів з БД, в show окремий post за id.
Для цих дій також створим шляхи (routes.rb).
resources :authors, only: %i[index show]
Після того нам потрібні відповідні представлення для дій index та show. Виводим список авторів на сторінці index.
Виводим список авторів на сторінці index.
# app/views/authors/index.html.slimh1 Authors= render partial: 'author', collection: @authors= link_to 'Усі публікації', posts_path
# app/views/authors/_author.html.slim.author h1 = author.name = link_to 'Переглянути', author_path(author.id)
У представленні show.html.slim, ми можем отримати доступ до окремого поста.
# app/views/authors/show.html.slimh1 = @author.name- if @posts h3 Список постів автора = render partial: 'posts/post', collection: @posts= link_to 'Повернутись назад', authors_path
Також давайте забезпечим можливість на сторінці posts/index.html.slim перейти на сторінку authors/index.html.slim
# app/views/posts/index.html.slim...= link_to 'Усі автори', authors_path
Забезпечим можливість переходу на сторінку автора зі сторінки поста.
# app/views/posts/show.html.slimh1 = @post.titlep = @post.descriptionspan Автор: = link_to @post.author.name, author_path(@post.author_id)br= link_to 'Усі публікації', posts_path
Отож, це одне з рішень нашої задачі, можливо ви вирішили її іншими способами, якщо ви знаєте як краще реалізувати певні пункти нашого завдання, то пишіть у коментарі.