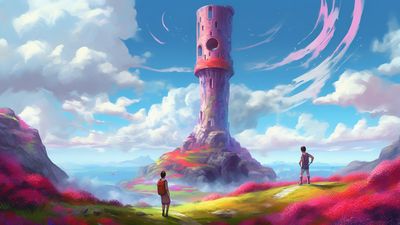
View (Practice) | Ruby on Rails
Practical task for the topic in the View
The first step in working with a model in Rails is to create it. For this
command line generator is used:
rails generate model Question
After that, we go to the project, to our db/migrate/ folder. We open ours
file with migration, where we will write which fields we want to be in our question.
class CreateQuestions < ActiveRecord::Migration[7.0]
def change
create_table :questions do |t|
t.string :title
t.text :body
t.references
t.timestamps
end
end
end
After creating the model, we need to run the migration with a special command to create the corresponding table in the database:
rake db:migrate
Let's repeat similar actions, but now for the Answer model with other fields.
class CreateAnswers < ActiveRecord::Migration[7.0]
def change
create_table :answers do |t|
t.text :content
t.references :question, foreign_key: true
t.timestamps
end
end
end
In Ruby on Rails, the references keyword is used in the context of migrations to define a relationship with another model. When you use references in a migration, it automatically creates a column in the database table to represent the relationship to another model and sets a foreign key to that column.
Let's now establish our connection using the has_many method in one
model and belongs_to method in another:
class Answer < ApplicationRecord
belongs_to :question
end
class Question < ApplicationRecord
has_many :answers
end
Logically, it is clear that a question can have several answers, and an answer can
belong to one issue. We cannot say that the answer fits to
several questions, that's why we use the connection "one to many".
It is important to add validation to the model to check that the input is correct before saving it to the database. For example, you can check whether the field is not empty and, under the condition of the task, the number of characters in the fields (Question model) is at least 35:
validates :title, presence: true, length: { minimum: 35 }
validates :body, presence: true, length: { minimum: 35 }
Validation for the model Answer:
validates :content, presence: true, length: { minimum: 50 }
Let's now use the after_save callback, which is executed after saving
models:
class Answer < ApplicationRecord
after_save :sanitize_content
belongs_to :question
validates :content, presence: true, length: { minimum: 35 }
private
def sanitize_content
self.content = filter_text(self.content)
self.save if self.changed?
end
def filter_text(text)
forbidden_words = %w[недоумок дурак]
forbidden_words.each do |word|
text = text.gsub(/\b#{word}\b/i, '***')
end
text
end
end
We've created a sanitize_content method where we update the content of our response. We also have another filter_text() method that is executed in the sanitize_content method. This is done for better code readability. In this method, we execute logic that replaces our "profanity" with "***". After that, we save the content (self.save) while checking that we somehow changed our response - self.changed?.
So, this is one of the solutions to our problem, maybe you solved it in other ways, if you know how to better implement certain points of our problem, then write in the comments.